Move data to workbooks
This article demonstrates a VBA macro that saves user input to a given workbook and worksheet programmatically. Macros are great for doing repetitive tasks, this saves you the steps to open a workbook, select a worksheet, save values to cells and lastly save the workbook and close.
This article demonstrates how to programmatically:
- Open a workbook based on a workbook name saved in a specific cell.
- Populate empty cells in the opened workbook with values.
- Create a new workbook if the workbook name doesn't exist.
- Insert a new worksheet if non-existing.
- Save changes
What's on this page
How the macro works
The Excel user enters a name, country, company, workbook, and worksheet name in cell range B3:F3. Then press the "Save record" button, see image above.
The macro tries to open the workbook in the same folder and copies cell range B3:F3 to the first empty row on the sheet. It then closes and saves the workbook.
If the workbook file can't be found it creates a new workbook and copies cell range B3:F3 to the first empty row on the sheet.
If the worksheet is not found the macros insert a new sheet and renames it. Then copies cell range B3:F3 to the first empty row.
Here is a flow chart.
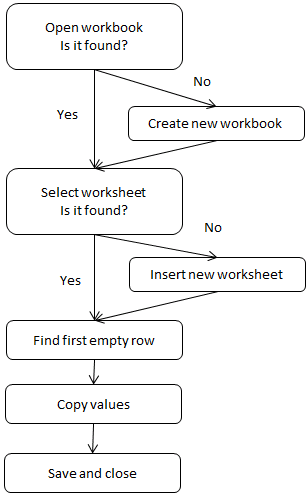
VBA code
'Name macro Sub Move_record_to_workbook() 'Save active workbook name to variable Wbs Wbs = ActiveWorkbook.Name 'Save value in cell F3 to variable WSName WSName = Range("F3").Value 'Save value in cell E3 to variable WBName WBName = Range("E3").Value 'Enable error handling On Error Resume Next 'Open workbook using path of active workbook and string in variable WBName Workbooks.Open Filename:=Application.ActiveWorkbook.Path & "\" & WBName 'Check if an arror has occurred If Err > 0 Then 'Disable error handling On Error GoTo 0 'Insert a new worksheet Workbooks.Add 'Enable error handling On Error Resume Next 'Activate worksheet WSName ActiveWorkbook.Worksheets(WSName).Activate 'Check if an arror has occurred, if so insert a new worksheet If Err > 0 Then Worksheets.Add(After:=Worksheets(1)).Name = WSName 'Disable error handling On Error GoTo 0 End If 'The With ... End With statement allows you to write shorter code by referring to an object only once instead of using it with each property. With ActiveWorkbook.Worksheets(WSName) 'Save row of first empty cell in column A to variable Lrow Lrow = .Range("A" & Rows.Count).End(xlUp).Row 'Check if cell value is not equal to nothing, if so add 1 to variable Lrow If .Range("A" & Lrow).Value <> "" Then Lrow = Lrow + 1 End With 'Copy values in cell range B3:F3, Sheet1 to a row in worksheet WSName based on row number in variable Lrow ActiveWorkbook.Worksheets(WSName).Range("A" & Lrow & ":C" & Lrow) = Workbooks(Wbs).Worksheets("Sheet1").Range("B3:F3").Value 'Save and close Workbook ActiveWorkbook.Close SaveChanges:=True End Sub
Where to put the macro?
- Press shortcut keys Alt+F11 to open the Visual Basic Editor.
- Press with mouse on "Insert" on the top menu.
- Press with left mouse button on "Module" to insert a new module to your workbook.
- Paste VBA code to window.
- Exit VB Editor and return to Excel.
How to link macro to a button?
- Go to tab "Developer". If tab "Developer" is missing.
- Press with left mouse button on the "Insert" button on the ribbon.
- Another menu shows up. Press with left mouse button on the button (Form Controls).
- Press and hold with left mouse button on worksheet where you want to place the button.
- Drag with mouse to size the button.
- Release left mouse button.
- A dialog box appears. Press with left mouse button on macro name "Move_record_to_workbook" to select it.
- Press with left mouse button on OK button to assign macro "Move_record_to_workbook" to the button.
You can move and resize the button using the handles if you are not happy with the location or size.
Files and folders category
Today I would like to share a macro that compares the content in two different folders and their subfolders. It […]
I will in this article demonstrate a macro that automatically opens all workbooks in a folder and subfolders, one by […]
This is a follow up to my last post Compare file names in two different folder locations and their subfolders, the obvious […]
Excel categories
3 Responses to “Move data to workbooks”
Leave a Reply
How to comment
How to add a formula to your comment
<code>Insert your formula here.</code>
Convert less than and larger than signs
Use html character entities instead of less than and larger than signs.
< becomes < and > becomes >
How to add VBA code to your comment
[vb 1="vbnet" language=","]
Put your VBA code here.
[/vb]
How to add a picture to your comment:
Upload picture to postimage.org or imgur
Paste image link to your comment.
=INDEX($B$1:$B$5, MATCH(0, COUNTIF($C$1:C1, $B$1:$B$5)+COUNTIF($A$1:$A$5, $B$1:$B$5), 0))
Oscar, you had given me this formula show an unique list for items in one column but not another.
How would you figure out an unique list where the sum of in one column doesn't match the other column? I'm comparing list 1 to list 2
List 1
A 10
A 10
B 5
B 6
B 4
List 2
A 10
A 10
B 5
B 6
Answer is B.
Oscar, I don't think there is a way to get an unique list comparing the sum of two lists.
[…] Sean asks: […]